[Javascript] Textareaなどの入力文字で、選択文字の入れ替えをするテクニック
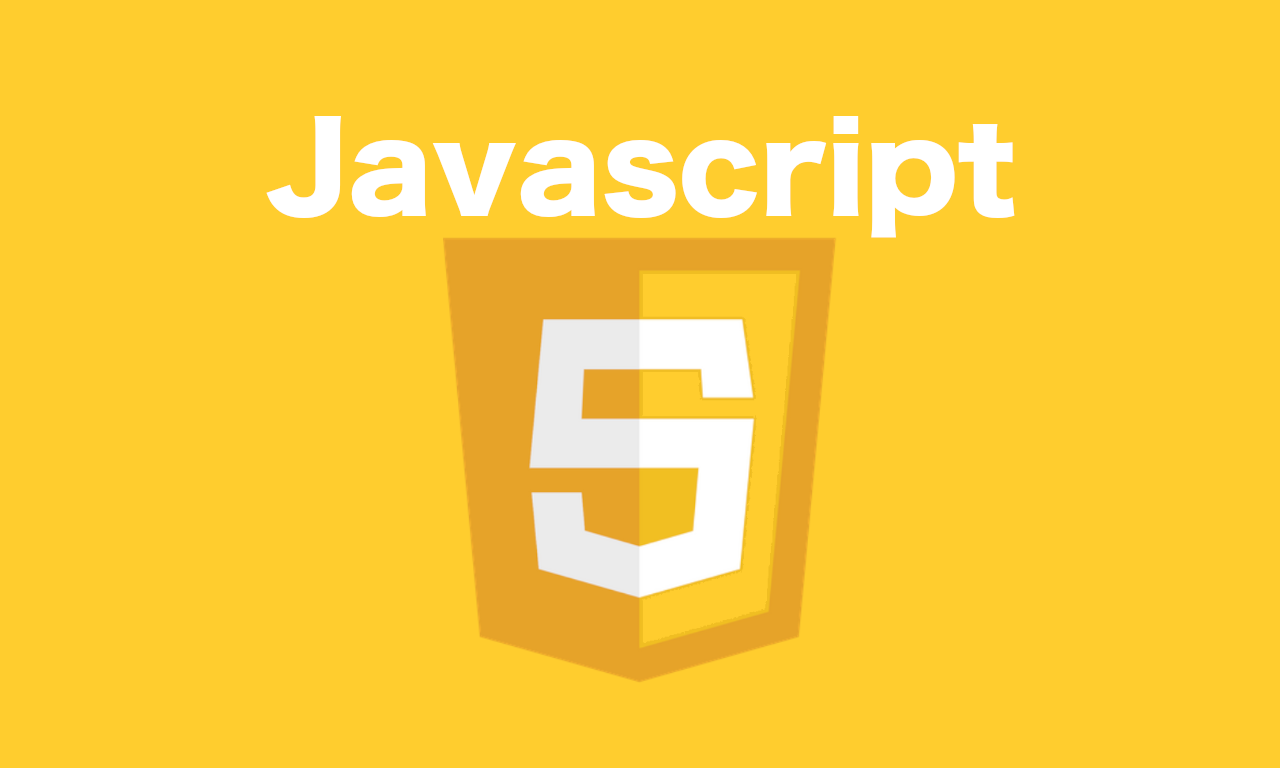
ソースコード
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset='utf-8' />
<title>Convert Tag</title>
<link rel='stylesheet' href='style.css' />
<script src='convert_tag.js'></script>
</head>
<body>
<textarea id='html'>古池や蛙飛こむ水のおと</textarea>
<blockquote id='converted'></blockquote>
<button id='b'>Bold</button>
<div>※文章を選択して、Boldボタンを押してください。</div>
</body>
</html>
style.css
html,body{
width : 100%;
height : 100%;
padding : 0;
margin : 0;
border : 0;
outline : 0;
scroll-behavior : smooth;
}
body{
padding:10px;
}
*, *:before, *:after {
-webkit-box-sizing : border-box;
-moz-box-sizing : border-box;
-o-box-sizing : border-box;
-ms-box-sizing : border-box;
box-sizing : border-box;
font-size:14px;
}
#html{
width:100%;
height:100px;
border:1px solid black;
padding:10px;
border-radius:8px;
outline:0;
}
#converted{
width:100%;
height:100px;
padding:10px;
border:1px dotted black;
border-radius:8px;
margin:0;
white-space:pre-wrap;
word-break:break-all;
}
convert_tag.js
(function(){
function Main(){
this.set_event()
new Convert()
}
Main.prototype.set_event = function(){
document.querySelector(`button#b`).addEventListener('click' , this.click_button.bind(this))
document.getElementById(`html`).addEventListener('input' , this.input.bind(this))
}
Main.prototype.click_button = function(){
const elm = document.getElementById('html') // 入力項目
const text = elm.value // 入力文字
const len = text.length //文字全体のサイズ
const start = elm.selectionStart //選択している開始位置
const end = elm.selectionEnd //選択している終了位置
const select = text.substr(start, end - start) //選択文字列
const before = text.substr(0, start) // 選択の前方文字列
const after = text.substr(end, len) // 選択の後方文字列
const word = `<b>${select}</b>` // 選択されている文字にbタグを追加
elm.value = before + word + after
elm.setSelectionRange(len, start + 1)
new Convert()
}
Main.prototype.input = function(){
new Convert()
}
function Convert(){
const html = document.getElementById('html')
const converted = document.getElementById('converted')
converted.innerHTML = html.value
}
switch(document.readyState){
case 'complete':
case 'interactive':
new Main()
return
default:
window.addEventListener('DOMContentLoaded' , (()=>{new Main()}))
}
})()
デモ
※文章を選択して、Boldボタンを押してください。